Hamburger Menu Animation with CSS!

Are you ready to transform your website's navigation with a captivating hamburger menu animation? Look no further! In this tutorial, we'll show you how to create a stunning hamburger menu using only CSS, no JavaScript required.
Start by creating a basic HTML structure with a container div, hamburger menu icon, and a hidden menu items section. Customize the menu items to suit your website's navigation needs.
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>Create a Stylish Hamburger Menu Animation with CSS!</title>
</head>
<body>
<div class="container">
<div id="hamburger">
<span></span>
<span></span>
<span></span>
<span></span>
<span></span>
<span></span>
</div>
<div class="hamburger-items">
<ul>
<li>
<a href="">
<div class="text">
<span></span><span></span>
</div>
</a>
</li>
<!-- Add more menu items here -->
</ul>
</div>
<!-- Dummy Content for Visualization -->
<section class="dummy-content">
<div class="circle"></div>
<div class="text">
<span></span><span></span>
</div>
<div class="square-top"></div>
<div class="square-behind"></div>
</section>
</div>
</body>
</html>
Copy and paste the provided CSS styles into your stylesheet. These styles define the appearance and animation of the hamburger menu icon and menu items. Feel free to customize the colors, sizes, and animation effects to match your website's design.
#hamburger {
width: 36px;
height: 36px;
position: relative;
margin: 20px;
-webkit-transform: rotate(0deg);
-moz-transform: rotate(0deg);
-o-transform: rotate(0deg);
transform: rotate(0deg);
-webkit-transition: .5s ease-in-out;
-moz-transition: .5s ease-in-out;
-o-transition: .5s ease-in-out;
transition: .5s ease-in-out;
cursor: pointer;
z-index: 999;
}
#hamburger span {
display: block;
position: absolute;
height: 3px;
width: 50%;
background: #d3531a;
opacity: 1;
-webkit-transform: rotate(0deg);
-moz-transform: rotate(0deg);
-o-transform: rotate(0deg);
transform: rotate(0deg);
-webkit-transition: .25s ease-in-out;
-moz-transition: .25s ease-in-out;
-o-transition: .25s ease-in-out;
transition: .25s ease-in-out;
}
#hamburger span:nth-child(even) {
left: 50%;
border-radius: 0 6px 6px 0;
}
#hamburger span:nth-child(odd) {
left: 0px;
border-radius: 6px 0 0 6px;
}
#hamburger span:nth-child(1),
#hamburger span:nth-child(2) {
top: 0px;
}
#hamburger span:nth-child(3),
#hamburger span:nth-child(4) {
top: 9px;
}
#hamburger span:nth-child(5),
#hamburger span:nth-child(6) {
top: 18px;
}
#hamburger.open span:nth-child(1),
#hamburger.open span:nth-child(6) {
-webkit-transform: rotate(45deg);
-moz-transform: rotate(45deg);
-o-transform: rotate(45deg);
transform: rotate(45deg);
}
#hamburger.open span:nth-child(2),
#hamburger.open span:nth-child(5) {
-webkit-transform: rotate(-45deg);
-moz-transform: rotate(-45deg);
-o-transform: rotate(-45deg);
transform: rotate(-45deg);
}
#hamburger.open span:nth-child(1) {
left: 3px;
top: 3px;
}
#hamburger.open span:nth-child(2) {
left: calc(50% - 3px);
top: 3px;
}
#hamburger.open span:nth-child(3) {
left: -50%;
opacity: 0;
}
#hamburger.open span:nth-child(4) {
left: 100%;
opacity: 0;
}
#hamburger.open span:nth-child(5) {
left: 3px;
top: 15px;
}
#hamburger.open span:nth-child(6) {
left: calc(50% - 3px);
top: 15px;
}
/_ Dummy Styles for Visualization _/
html,
body {
margin: 0;
padding: 0;
}
.container {
position: relative;
width: 100%;
height: 100vh;
background-color: #533557;
overflow: hidden;
}
.dummy-content {
position: relative;
text-align: center;
transition: 0.5s;
transform: scale(1.2);
padding-top: 5rem;
}
.circle {
display: inline-block;
width: 75px;
height: 75px;
background-color: #EC7263;
border-radius: 100%;
}
.text {
margin: 15px 0 30px;
}
.text span {
display: inline-block;
height: 10px;
margin: 0 5px;
background-color: #C06162;
border-radius: 5px;
}
.text span:first-child {
width: 50px;
}
.text span:last-child {
width: 80px;
}
.square-top {
display: inline-block;
position: relative;
width: 200px;
height: 300px;
background-color: #FEBE7E;
z-index: 1;
}
.square-behind {
display: inline-block;
position: relative;
top: -256px;
width: 250px;
height: 210px;
background-color: #C28683;
}
.square-behind:before,
.square-behind:after {
position: absolute;
content: '';
top: 0;
width: 40%;
height: 100%;
}
.square-behind:before {
left: 0;
background-color: #9D567C;
}
.square-behind:after {
right: 0;
background-color: #958C6B;
}
.hamburger-items {
position: absolute;
left: -100%;
transition: all ease 0.2s;
background-color: #3d2341;
width: 90%;
height: 100vh;
top: 0;
z-index: 99;
padding-top: 6rem;
}
.hamburger-items ul li {
list-style: none;
}
.hamburger-items ul li .text span:first-child {
width: 30px;
}
.hamburger-items ul li .text span:last-child {
width: 150px;
}
.hamburger-items.show {
left: 0;
transition: all ease 0.2s;
}
The hamburger menu animation can be toggled open and closed using JavaScript. We've included a simple script using jQuery to toggle the "open" class on click, revealing or hiding the menu items. You can adjust this functionality as needed or implement your own custom JavaScript solution.
$(document).ready(function () {
$('#hamburger').click(function () {
$(this).toggleClass('open');
$(".hamburger-items").toggleClass("show");
});
});
With just a few lines of CSS, you can create a visually stunning hamburger menu animation that will impress your website visitors. Experiment with different styles and effects to find the perfect design for your website's navigation. Let your creativity shine and elevate your user experience with this stylish hamburger menu!
And here is the result:
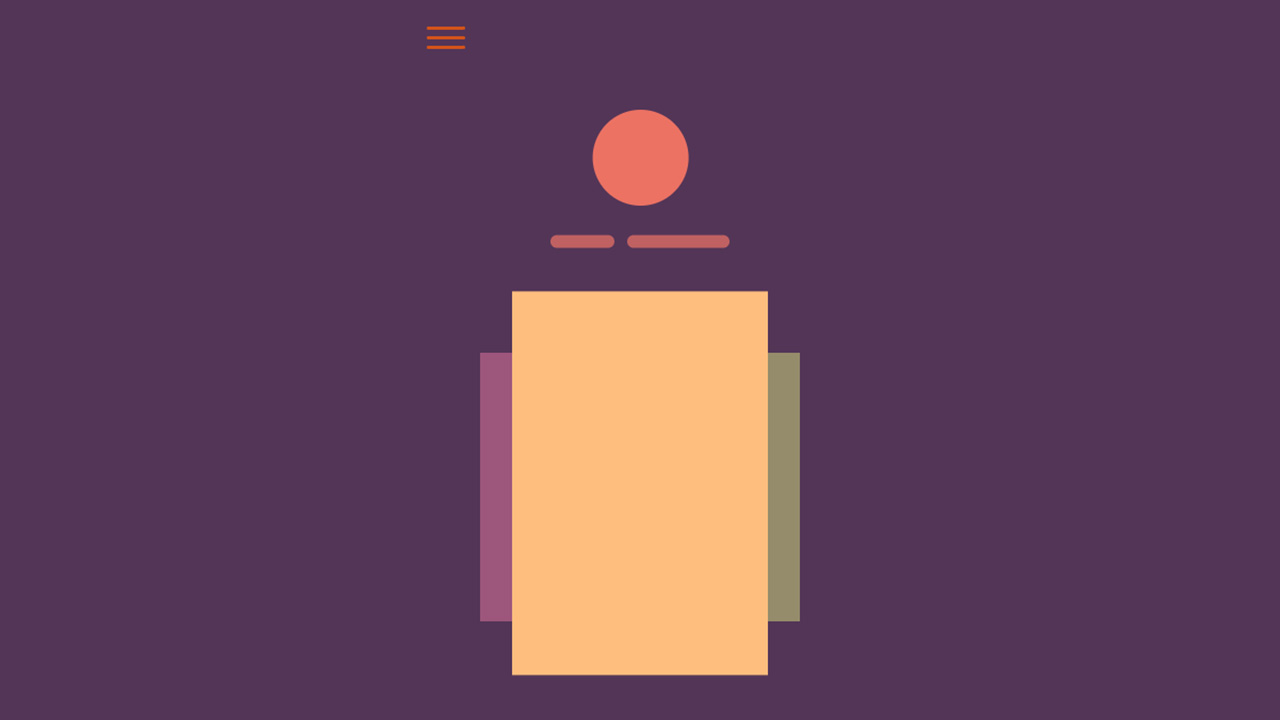
See the full video:
- CSS
- CSS3 Animation
- Front End Development
- Mobile Menu
- Hamburger Menu
- Hamburger Menu Animation
- CSS Animation