Mastering Series: CSS Styling
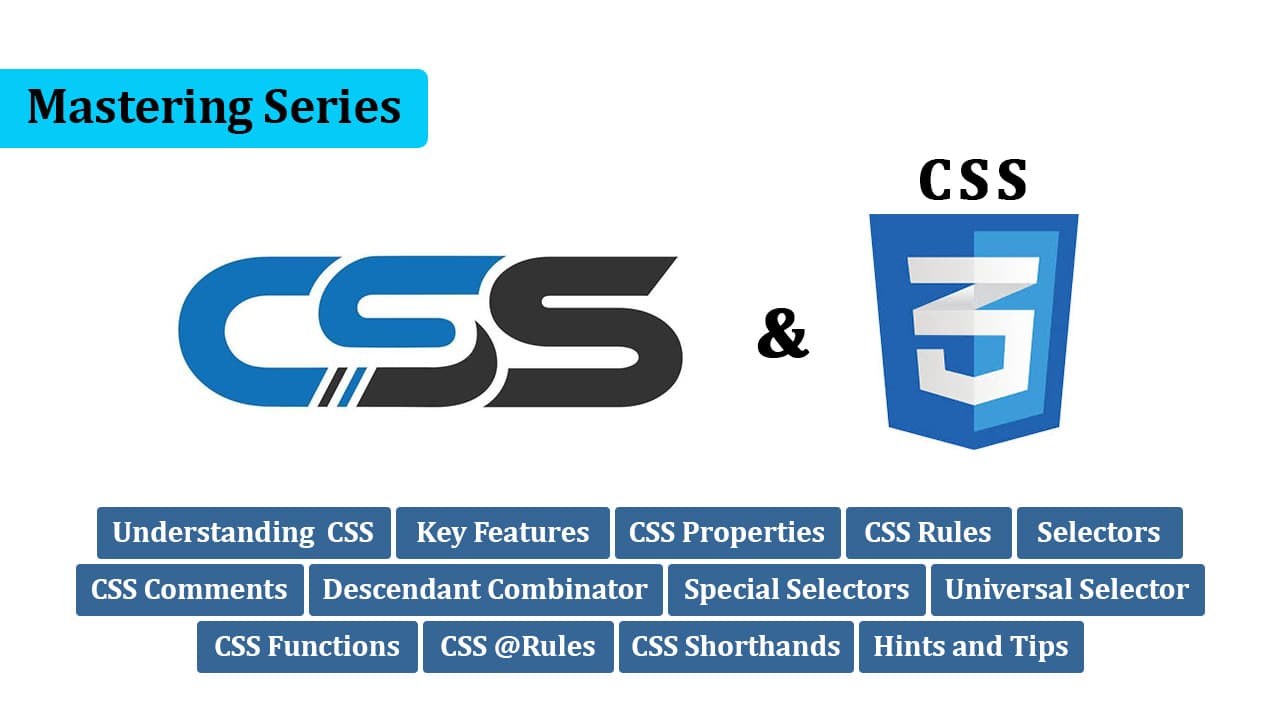
Just like our previous course focused on Mastering HTML, this course is exclusively dedicated to Mastering CSS. Here, we cover CSS comprehensively.
Before diving into CSS, we explored the formatting and structure of HTML and CSS in our previous courses. We discussed how these languages work and their roles in shaping web pages.
CSS, short for Cascading Style Sheets, is a language learned after HTML. It serves as the styling tool for HTML documents. If you're unfamiliar with HTML, we recommend reading our HTML article here before proceeding further.
CSS complements HTML by defining the visual presentation of web content. It allows developers to set fonts, colors, spacing, layouts, and animations, thereby enhancing the user interface.
- CSS is part of the web standards technologies, alongside HTML and JavaScript, defined by organizations like W3C, WHATWG, ECMA, or Khronos.
- Despite constant updates, CSS remains backward-compatible, ensuring older websites maintain their functionality.
- CSS enables developers to customize HTML elements for various devices and screen sizes, including computers, paper (print), and mobile devices.
CSS offers numerous properties to style HTML elements, such as color
, font-size
, background-color
, margin
, padding
, border
, and more.
CSS operates on a rule-based system, allowing developers to define styling rules for specific HTML elements using selectors. For example:
h1 {
color: blue;
font-size: 30px;
}
Here, h1
serves as the selector, and we apply styles like color
and font-size
.
Let's explore CSS in action by previewing its effects on HTML elements.
Before we begin, let's open our HTML file in an editor and a web browser to preview our changes.
=> Types of CSS Implementation with Preview
- Inline CSS: Applying CSS directly within HTML elements
<h1 style="color: blue; font-size: 30px;">Hello, World!</h1>
- Internal CSS: Embedding CSS within the
<style>
tag in the HTML document.
<style>
h1 {
color: blue;
font-size: 30px;
}
</style>
<h1>Hello, World!</h1>
- External CSS: Linking an external CSS file to the HTML document.
<!-- index.html -->
<link rel="stylesheet" type="text/css" href="styles.css">
<h1>Hello, World!</h1>
/* styles.css */
h1 {
color: blue;
font-size: 30px;
}
Learning CSS is straightforward. You just need to understand its properties and their uses. As you encounter different layouts and elements, you'll intuitively know how to style them with CSS.
color
: Used to set the text color.
p {
color: red;
}
font-size
: Used to set the size of the font.
h1 {
font-size: 24px;
}
background-color
: Used to set the background color.
body {
background-color: #f0f0f0;
}
margin
: Used to create space around an element.
.container {
margin: 10px;
}
padding
: Used to create space within an element.
.box {
padding: 20px;
}
border
: Used to create a border around an element.
.image {
border: 1px solid #ccc;
}
width
: Set width of an element.
.container {
width: 80%;
}
list-style
: Set list style of the list.
ul {
list-style: circle;
}
background-image
: Used to set background images or with linear gradient.
.hero-section {
background-image: linear-gradient(to right, #ff8a00, #da1b60);
background-image: url("./images/hero-image.png");
}
opacity
: Used to set alpha opacity of an element.
.overlay {
background-color: black;
opacity: 0.5;
}
position
: Used to set the position of the element.
.tooltip {
position: absolute;
top: 50px;
left: 20px;
}
display
: Used to specify the display behavior of an element.
.inline-block {
display: inline-block;
}
These examples illustrate how each property can be used to style different elements on a webpage, providing versatility and control over the design and layout.
We can give styling to any element of HTML in 3 ways
- By Selector: If we have taken any default tag of HTML to give us style.. That is… H1, p, span, div, ...
<h1>This is heading</h1>
<p>This is paragraph</p>
<span>This is span element</span>
<div>This is div element</div>
h1, p, span, div {
color: blue;
}
- By ID: If there are many similar elements in your HTML page then you can style that particular element by differentiating it by using an ID.
<div id="unique-element">This is div element</div>
#unique-element {
font-size: 18px;
}
- By Class: Just like we told for ID that by looking at your ID we can style you, in the same way we can style you by giving class. The only difference between ID and Class is that we can write ID only once in a page and Class is different.
<div class="highlight">This is div element</div>
.highlight {
background-color: yellow;
}
These selectors provide flexibility in applying styles to specific elements, allowing for targeted styling based on element type, unique identifiers (IDs), or shared characteristics (classes).
In the vast expanse of CSS, comments serve as more than just silent observers—they're the guiding lights illuminating the path to clarity and comprehension.
Imagine yourself navigating through a labyrinth of styles, each line a potential fork in the road. Without comments, it's like stumbling through the dark, unsure of where each twist and turn might lead. But with comments as your guide, the journey becomes clearer, each annotation a signpost marking your progress.
Let's take a moment to appreciate the power of comments in action:
/* This comment explains the purpose of the following styles */
.navigation {
/* Set navigation styles here */
}
/* Uncomment this block to activate the alternate layout */
/*
.navigation {
/* Alternate navigation styles */
}
*/
/* TODO: Implement responsive design for mobile */
@media screen and (max-width: 768px) {
/* Mobile-specific styles */
}
See how comments provide context, offer alternative solutions, and even outline future tasks? They're like breadcrumbs leading you back home, ensuring you never lose your way in the maze of CSS.
So, next time you're crafting your CSS masterpiece, don't forget to sprinkle in a few comments along the way. Your future self—and your fellow developers—will thank you for the clarity you've brought to the code.
In the labyrinth of web development, CSS provides us with a powerful tool known as the descendant combinator. This combinator allows us to traverse the document tree and apply styles based on the relationship between elements.
Imagine you have an <article>
tag containing several <p>
tags within it. With the descendant combinator, you can easily target those <p>
tags specifically within the <article>
tag.
<article>
<p>Article paragraph</p>
<div>
<p>Article inner paragraph</p>
</div>
<p>Article sibling paragraph</p>
</article>
Let's explore how it works:
/* Style all <p> tags within <article> */
article p {
/* Styles for paragraphs within articles */
}
/* Target <p> directly inside <article> */
article > p {
/* Styles for direct child paragraphs within articles */
}
/* Style <p> following <div> */
article div + p {
/* Styles for paragraphs immediately following div */
}
By using these combinators, you can precisely tailor your styles to specific elements and their relationships within the document structure. It's like having a GPS for your CSS, guiding you through the intricate paths of web design.
CSS offers a realm of special selectors known as pseudo-classes and pseudo-elements, allowing for fine-grained control over element styles based on various conditions and structural cues.
=> Pseudo-classes
:first-child
: Targets the first child element of its parent.:last-child
: Targets the last child element of its parent.:invalid
: Targets form elements that have failed validation.:hover
: Styles an element when the mouse hovers over it.:focus
: Styles an element when it gains focus.
/* Example of using :hover pseudo-class */
button:hover {
background-color: #3498db; /* Changes background color on hover */
}
It's essential to note that in CSS, class or id names should not start with a number; however, numbers can be included in the middle or end of the name.
=> Pseudo-elements
::first-line
: Styles the first line of a block-level element.::before
: Inserts content before the content of an element.::after
: Inserts content after the content of an element.
/* Example of using ::after pseudo-element */
.box::after {
content: "➥"; /* Adds arrow after content of .box */
}
/* Combination of pseudo-classes and pseudo-elements */
article p:first-child::first-line {
font-size: 120%;
font-weight: bold;
}
These special selectors empower developers to create dynamic and engaging user experiences, elevating the aesthetics and functionality of web pages.
In CSS, the universal selector, denoted by an asterisk (*), holds the power to target all elements within an HTML document. When used strategically, it offers a convenient way to apply styles universally.
/* Example of using the universal selector */
* {
margin: 0px; /* Applies margin reset to all elements */
}
By employing the universal selector, like in the example above, you can swiftly implement global styling adjustments across your entire webpage. However, exercise caution, as overly broad application may unintentionally override more specific styling rules.
In CSS, functions provide a way to introduce dynamicity into style declarations. While some values are straightforward keywords or numerical values, others utilize functions to calculate or transform properties.
/* Example of using calc() function */
width: calc(100% - 10px); /* Calculates the width dynamically */
/* Example of using rotate() function */
transform: rotate(0.8turn); /* Rotates an element by 0.8 turn */
By leveraging functions like calc()
and rotate()
, you can create more flexible and interactive designs, allowing for calculations and transformations that adapt to different contexts and user interactions.
In CSS, @rules
are directives that instruct the browser on how to interpret and apply styles. These rules define various aspects of styling, such as importing external stylesheets or applying styles conditionally based on certain criteria.
/* Example of @import rule */
@import "styles2.css"; /* Imports an external stylesheet */
/* Example of @media rule */
@media (min-width: 30em) {
body {
background-color: blue; /* Applies blue background when the minimum width is 30em */
}
}
With @rules
, you can manage your stylesheets more efficiently and create responsive designs that adapt to different devices and screen sizes.
In CSS, shorthand properties allow you to set multiple related properties using a single line of code. This helps to streamline your CSS code and make it more concise. Here are some commonly used shorthand properties:
=> Padding and Margin
Instead of specifying each side individually (padding-top, padding-right, padding-bottom, padding-left), you can use shorthand like padding: top right bottom left.
Example: padding: 20px 10px 0px 0px; (Top Right Bottom Left)
/* Individual padding */
.element {
padding-top: 20px;
padding-right: 10px;
padding-bottom: 0px;
padding-left: 0px;
}
/* Shorthand padding */
.element {
padding: 20px 10px 0px 0px; /* Top Right Bottom Left */
}
=> Font
The font
shorthand property allows you to set various font properties in a single declaration.
Format: font: font-style font-variant font-weight font-size/line-height font-family;
Example: font: italic small-caps bold 20px/30px Arial;
/* Individual font properties */
.element {
font-style: italic;
font-variant: small-caps;
font-weight: bold;
font-size: 20px;
line-height: 30px;
font-family: Arial;
}
/* Font shorthand */
.element {
font: italic small-caps bold 20px/30px Arial;
}
=> Background
The background
shorthand property lets you set multiple background properties in one declaration.
Format: background: background-color background-image background-position background-repeat background-attachment;
Example: background: red url("./xyz.jpg") 10px 10px repeat-x fixed;
/* Individual background properties */
.element {
background-color: red;
background-image: url("./xyz.jpg");
background-position: 10px 10px;
background-repeat: repeat-x;
background-attachment: fixed;
}
/* Background shorthand */
.element {
background: red url("./xyz.jpg") 10px 10px repeat-x fixed;
}
Using shorthand properties can make your CSS code more efficient and easier to read, especially when you need to set multiple related properties at once.
Just as in HTML, if we have any space, tabs, or any code written in a new line, the browser will ignore that white space. Similarly, in CSS also, if we have any white space, it will be ignored. CSS is only for us, so you can write it in a single line, put comments in between, or write it in a new line; you can do anything.
You can also write CSS in one line if you want. For example:
body {
font: 1em/150% Helvetica, Arial, sans-serif;
padding: 1em;
margin: 0 auto;
max-width: 33em;
}
/* OR */
body { font: 1em/150% Helvetica, Arial, sans-serif; padding: 1em; margin: 0 auto; max-width: 33em; }
And if you want, you can also write CSS in separate lines.
One thing we have to keep in mind is that if we have made any mistake in the spelling of properties or values in CSS, then it will not show us any error on the browser. To learn how to write correctly, we will focus on writing CSS correctly.
If you want to check whether your CSS is proper or if there are any mistakes in it, you can validate your CSS through the W3C CSS Validator. This tool helps you ensure that your CSS follows the standards.
Now, you need to think about all the properties of CSS and how they are used. I recommend referring to the W3Schools website to explore all the properties. By doing so, you can gain a better understanding of CSS and know which properties to use when styling your layouts.
See the full video:
- Front-End Development
- CSS
- Key Features of CSS
- CSS Properties
- CSS Rules
- CSS Types
- CSS Rules
- CSS Styling
- CSS Comments
- Descendant Combinator
- CSS Special Selectors
- Pseudo-classes
- Pseudo-elements
- Universal Selector
- Functions in CSS
- CSS @Rules
- CSS Shorthands
- CSS Hints
- CSS Tips