Mastering Series: jQuery
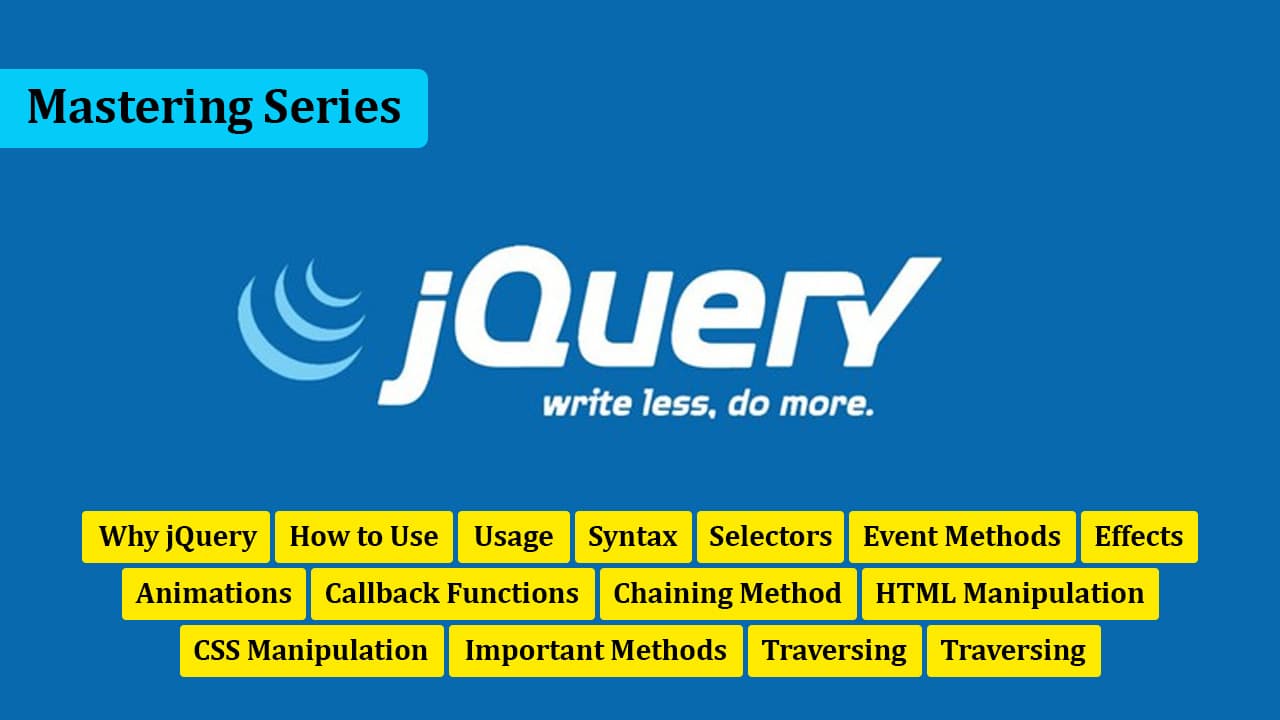
So far, we have seen basic and advanced HTML and CSS, and now we need to have some knowledge of JavaScript to start the project series in front-end development. In this video, we will understand jQuery: what it is, how it is used, and what we can do with it. We will cover everything in detail.
JavaScript is a very large language with a comprehensive library that might seem a bit difficult at first. So, for now, we will focus on learning the front-end, starting with jQuery, a simplified and lightweight JavaScript library.
jQuery is a popular, fast, and lightweight JavaScript library designed to simplify the client-side scripting of HTML. While JavaScript and jQuery share similarities, jQuery streamlines JavaScript by allowing us to perform complex tasks with less code.
jQuery is incredibly popular and extendable, making it a go-to choice for many web developers. Companies like Google, Microsoft, IBM, and Netflix utilize jQuery for their web projects. Here are some key features of jQuery:
- HTML/DOM Manipulation: Easily select and manipulate HTML elements and their attributes.
- CSS Manipulation: Apply and change CSS styles dynamically.
- HTML Event Methods: Simplify event handling, like clicks, hovers, etc.
- Effects and Animations: Create engaging animations and transitions.
- AJAX: Perform asynchronous HTTP requests.
- Utilities: Helpful functions for tasks like iteration and array manipulation.
jQuery can be added to your page in three ways:
=> Inline Script:
<head>
<a href="javascript:void(0)">About page</a>
</head>
=> Internal Script:
<head>
<script type="text/javascript">
Your script goes here
</script>
</head>
=> External Script:
<head>
<script type="text/javascript" src="functions.js"></script>
</head>
Adding jQuery to Your Project
To use jQuery, you need to add it to your web page. There are two primary methods: downloading the jQuery library or using a CDN (Content Delivery Network).
-
Download and Use jQuery Library
- Visit jquery.com and download either the production or development version.
- Production version: Minified and compressed for live websites.
- Development version: Uncompressed for testing and development.
- Include the downloaded file in your HTML:
<head> <script src="jquery-3.7.1.min.js"></script> </head>
-
Using jQuery CDN
- Add the following script tag to your HTML head to link to jQuery's CDN:
<head> <script src="https://ajax.googleapis.com/ajax/libs/jquery/3.7.1/jquery.min.js"></script> </head>
To illustrate how jQuery simplifies tasks, let's look at a basic example of how you might use jQuery to manipulate the DOM and handle an event:
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8" />
<meta name="viewport" content="width=device-width, initial-scale=1.0" />
<title>jQuery Example</title>
<script src="https://ajax.googleapis.com/ajax/libs/jquery/3.7.1/jquery.min.js"></script>
<script>
$(document).ready(function () {
$("button").click(function () {
$("p").toggle();
});
});
</script>
</head>
<body>
<button>Toggle Paragraph</button>
<p>This is a paragraph with jQuery.</p>
</body>
</html>
In this example, we use jQuery to toggle the visibility of a paragraph when a button is clicked. The $(document).ready
function ensures that the DOM is fully loaded before executing the script.
With jQuery, we can perform various actions by selecting our HTML elements. The basic syntax is designed to select HTML elements and perform actions on them.
-
Basic Syntax:
$(selector).action();
$
sign is used to access jQuery.(selector)
is used to select HTML elements..action()
is used to perform an action on the selected elements.
-
Examples:
$(this).hide(); // hides the current element. $("p").hide(); // hides all <p> elements. $(".test").hide(); // hides all elements with class="test". $("#test").hide(); // hides the element with id="test".
-
Document Ready Function: jQuery methods are written inside the document ready function to ensure they are executed after the page is fully loaded.
$(document).ready(function () { // jQuery methods go here... });
Or:
$(function () { // jQuery methods go here... });
The internal
<script>
can also be written inside the<body>
tag.
jQuery selectors are one of the most important parts of the jQuery library. Using jQuery selectors, we can find HTML elements by tag, class, id, attributes, and attribute values. This is based on CSS selectors, but jQuery also has some custom selectors.
-
Basic Selectors:
$("p"); // Selects all <p> elements. $(".heading"); // Selects all elements with class="heading". $("#layout"); // Selects the element with id="layout".
-
Universal Selector:
$("*"); // Selects all elements.
-
Contextual Selectors:
$(this); // Selects the current element. $("p:first"); // Selects the first <p> element. $("ul li:first"); // Selects the first <li> element within the first <ul>. $("ul li:first-child"); // Selects the first <li> child of each <ul>.
-
Attribute Selectors:
$("[href]"); // Selects all elements with an href attribute.
-
Form Selectors:
$(":button"); // Selects all <button> elements and input elements of type="button".
-
Even/Odd Selectors:
$("tr:even"); // Selects all even <tr> elements. $("tr:odd"); // Selects all odd <tr> elements.
What are events? jQuery is designed to respond to events on an HTML page. Events are actions that occur on the page, such as mousing over an element, selecting a radio item, or clicking on an element.
$("p").click(function () {
// action goes here!!
});
$(document).ready()
:
With this method, we can execute whatever functions we want to perform after our web page is completely loaded.click()
:
This method connects the event handler function to HTML elements. When the user clicks on the HTML element, the function is executed.$("p").click(function () { $(this).hide(); });
Or:
$("p").on("click", function () { $(this).hide(); });
dblclick()
:
Similar to the click method, but it performs the action on double-click.$("p").dblclick(function () { $(this).hide(); });
mouseenter()
:
Executes the function when the mouse cursor enters the HTML element.$("p").mouseenter(function () { alert("You entered p1!"); });
mouseleave()
:
Executes the function when the mouse cursor leaves the HTML element.$("p").mouseleave(function () { alert("Bye! You now leave p1!"); });
mousedown()
:
Executes the function when the mouse button is pressed down on the HTML element.$("#p1").mousedown(function () { alert("Mouse down over p1!"); });
mouseup()
:
Executes the function when the mouse button is released over the HTML element.$("#p1").mouseup(function () { alert("Mouse up over p1!"); });
hover()
:
Combines the functionality of mouseenter() and mouseleave().$("#p1").hover(function () { alert("You entered p1!"); });
focus()
:
Executes the function when an element gains focus.$("input").focus(function () { $(this).css("background-color", "#cccccc"); });
blur()
:
Executes the function when an element loses focus.$("input").blur(function () { $(this).css("background-color", "#ffffff"); });
- Multiple Events:
We can perform several events simultaneously using the on() method.$("p").on({ mouseenter: function () { $(this).css("background-color", "lightgray"); }, mouseleave: function () { $(this).css("background-color", "lightblue"); }, click: function () { $(this).css("background-color", "yellow"); }, });
With the help of jQuery, we can show or hide any elements, toggle them, or animate them with fade effects.
-
Show/Hide/Toggle:
$(selector).show(speed, callback); $(selector).hide(speed, callback); $(selector).toggle(speed, callback);
- Examples:
$("#hide").click(function(){ $("p").hide(); }); $("#show").click(function(){ $("p").show(); }); $("button").click(function(){ $("p").hide(1000); }); $("button").click(function(){ $("p").toggle(); });
-
Fade Effects:
$(selector).fadeIn(speed, callback); $(selector).fadeOut(speed, callback); $(selector).fadeToggle(speed, callback); $(selector).fadeTo(speed, opacity, callback);
- Examples:
$("#flip").click(function(){ $("#panel").slideDown(); }); $("#flip").click(function(){ $("#panel").slideUp(); }); $("#flip").click(function(){ $("#panel").slideToggle(); });
-
Animate:
$(selector).animate({ params }, speed, callback);
- Examples:
$("button").click(function(){ $("div").animate({left: '250px'}); }); $("button").click(function(){ $("div").animate({ left: '250px', opacity: '0.5', height: '150px', width: '150px' }); }); $("button").click(function(){ $("div").animate({ left: '250px', height: '+=150px', width: '+=150px' }); }); $("button").click(function(){ $("div").animate({ height: 'toggle' }); }); $("button").click(function(){ var div = $("div"); div.animate({height: '300px', opacity: '0.4'}, "slow"); div.animate({width: '300px', opacity: '0.8'}, "slow"); div.animate({height: '100px', opacity: '0.4'}, "slow"); div.animate({width: '100px', opacity: '0.8'}, "slow"); }); $("#stop").click(function(){ $("#panel").stop(); });
Callback functions in jQuery are executed after a specific action or effect has been applied to HTML elements and its performance is complete.
In jQuery, just like in JavaScript, statements are executed sequentially from top to bottom. However, when applying effects or actions to HTML elements, the effect is executed first, and then the callback function is triggered upon completion.
$("button").click(function () {
$("p").hide("slow", function () {
alert("The paragraph is now hidden");
});
});
In this example:
- When a button is clicked, the
<p>
elements on the page are hidden slowly. - Once the hiding effect is complete, the callback function is triggered, displaying an alert message stating "The paragraph is now hidden".
Callback functions are useful for executing additional actions or logic after completing an effect or action on HTML elements. They provide a way to control the flow of code execution and perform tasks synchronously with the applied effects.
Chaining in jQuery allows us to apply multiple methods or effects to the same set of elements in a single statement. This simplifies our code and improves readability by reducing the number of lines required.
$("#p1").css("color", "red").slideUp(2000).slideDown(2000);
In this example:
- The
<p>
element with the IDp1
is selected. - The
.css()
method is applied to change the color of the text to red. - The
.slideUp()
method is then applied to hide the element with a sliding motion over a duration of 2000 milliseconds (2 seconds). - Finally, the
.slideDown()
method is applied to show the element again with a sliding motion over the same duration.
In jQuery, HTML manipulation allows us to dynamically change the content and attributes of HTML elements on our webpage. We can retrieve text, HTML content, and attribute values, as well as set new values or add new elements to the DOM.
Retrieving Content:
To retrieve text content of an element:
$("#btn1").click(function () {
alert("Text: " + $("#test").text());
});
To retrieve HTML content of an element:
$("#btn2").click(function () {
alert("HTML: " + $("#test").html());
});
To retrieve value of an input field:
$("#btn1").click(function () {
alert("Value: " + $("#test").val());
});
To retrieve the value of an attribute:
$("button").click(function () {
alert($("#w3s").attr("href"));
});
Setting Content:
To set text content of an element:
$("#test1").text("Hello world!");
To set HTML content of an element:
$("#test2").html("<b>Hello world!</b>");
To set value of an input field:
$("#test3").val("Dolly Duck");
Callback Function with text() and html()
Using a callback function with text() method:
$("#test1").text(function (i, origText) {
return "Old text: " + origText + " New text: Hello world! (index: " + i + ")";
});
Using a callback function with html() method:
$("#test2").html(function (i, origText) {
return (
"Old html: " +
origText +
" New html: Hello <b>world!</b> (index: " +
i +
")"
);
});
Manipulating Attributes
To change the value of an attribute:
$("button").click(function () {
$("#w3s").attr("href", "https://www.w3schools.com/jquery/");
});
To change multiple attributes simultaneously:
$("button").click(function () {
$("#w3s").attr({
href: "https://www.w3schools.com/jquery/",
title: "W3Schools jQuery Tutorial",
});
});
Adding and Removing Elements
To add new elements:
$("p").append("Some appended text."); // Appends text to the end
$("p").prepend("Some prepended text."); // Prepends text to the beginning
$("img").after("Some text after"); // Adds text after the element
$("img").before("Some text before"); // Adds text before the element
To remove elements:
$("#div1").remove(); // Removes the selected element
$("#div1").empty(); // Removes all child elements and text from the selected element
HTML manipulation in jQuery provides powerful tools for dynamically updating and modifying content on our webpage, enhancing user interaction and experience.
Just as we can manipulate HTML elements dynamically with jQuery, we can also manipulate their styles. With CSS manipulation, we can dynamically add, remove, or toggle classes, as well as directly apply CSS styles to elements.
Adding a Class
To add a class to selected elements:
$("button").click(function () {
$("h1, h2, p").addClass("blue");
$("div").addClass("important");
});
Removing a Class
To remove a class from selected elements:
$("button").click(function () {
$("h1, h2, p").removeClass("blue");
});
Toggling a Class
To toggle a class on selected elements:
$("button").click(function () {
$("h1, h2, p").toggleClass("blue");
});
Applying Inline Styles
To apply inline styles using the css() method:
$("p").css("background-color", "yellow");
The syntax is: css("propertyname", "value")
.
Applying Multiple Styles
To apply multiple styles at once:
$("p").css({ "background-color": "yellow", "font-size": "200%" });
CSS manipulation in jQuery allows us to dynamically control the appearance of our elements, enhancing visual presentation and user experience.
In jQuery, there are several important methods available for working with dimensions:
width()
height()
innerWidth()
innerHeight()
outerWidth()
outerHeight()
These methods allow us to retrieve or set the width and height of elements. However, it's important to note that border, padding, and margin are handled differently depending on the method used.
Retrieving Width and Height
To retrieve the width and height of an element using width()
and height()
:
$("button").click(function () {
var txt = "";
txt += "Width: " + $("#div1").width() + "</br>";
txt += "Height: " + $("#div1").height();
$("#div1").html(txt);
});
Inner Width and Height
The innerWidth()
and innerHeight()
methods include padding but exclude border and margin:
$("button").click(function () {
var txt = "";
txt += "Inner width: " + $("#div1").innerWidth() + "</br>";
txt += "Inner height: " + $("#div1").innerHeight();
$("#div1").html(txt);
});
Outer Width and Height
The outerWidth()
and outerHeight()
methods include padding and border, but not margin:
$("button").click(function () {
var txt = "";
txt += "Outer width: " + $("#div1").outerWidth() + "</br>";
txt += "Outer height: " + $("#div1").outerHeight();
$("#div1").html(txt);
});
Including Margin
To include margin in the calculation, use outerWidth(true)
and outerHeight(true)
:
$("button").click(function () {
var txt = "";
txt += "Outer width (+margin): " + $("#div1").outerWidth(true) + "</br>";
txt += "Outer height (+margin): " + $("#div1").outerHeight(true);
$("#div1").html(txt);
});
These methods provide precise control over element dimensions, allowing for accurate layout and positioning in your web applications.
Traversing in jQuery refers to the process of navigating through the DOM (Document Object Model) to find or select other HTML elements based on their relationship to each other. Understanding traversing is essential for efficient manipulation and interaction with the DOM tree.
Parent Elements
-
parent()
:
Retrieves the direct parent element of the selected element.$(document).ready(function () { $("span").parent(); });
-
parents()
:
Retrieves all parent elements of the selected elements up to the<html>
tag.$(document).ready(function () { $("span").parents(); });
-
parentsUntil()
:
Retrieves all parent elements of the selected elements up to but not including the element specified as an argument.$(document).ready(function () { $("span").parentsUntil("div"); });
Child Elements
-
children()
:
Retrieves the direct child elements of the selected elements.$(document).ready(function () { $("div").children(); });
-
children()
with filter:
Filters child elements based on the specified argument.$(document).ready(function () { $("div").children("p.first"); });
Descendant Elements
find()
:
Searches for descendant elements based on the specified argument.$(document).ready(function () { $("div").find("span"); });
Sibling Elements
-
siblings()
:
Retrieves all sibling elements of the selected elements.$(document).ready(function () { $("h2").siblings(); });
-
siblings()
with filter:
Filters sibling elements based on the specified argument.$(document).ready(function () { $("h2").siblings("p"); });
Next and Previous Elements
-
next()
:
Retrieves the next sibling element of the selected elements.$(document).ready(function () { $("h2").next(); });
-
nextAll()
:
Retrieves all sibling elements that come after the selected elements.$(document).ready(function () { $("h2").nextAll(); });
-
nextUntil()
:
Retrieves all sibling elements between the selected elements and the specified argument.$(document).ready(function () { $("div").nextUntil("h6"); });
Filtering Methods
first()
: Retrieves the first element from the selected elements.last()
: Retrieves the last element from the selected elements.eq()
: Retrieves the element at the specified index from the selected elements.$(document).ready(function () { $("p").eq(1); });
filter()
: Filters elements based on the specified criteria.$(document).ready(function () { $("p").filter(".intro"); });
not()
: Excludes elements that match the specified criteria.$(document).ready(function () { $("p").not(".intro"); });
Understanding jQuery traversing methods enables precise selection and manipulation of DOM elements, enhancing the functionality and interactivity of web pages.
Congratulations on completing the Mastering jQuery course! Throughout this journey, you've delved deep into the world of jQuery, a powerful JavaScript library that simplifies DOM manipulation, event handling, animation, and more. Let's recap some key takeaways:
- Understanding jQuery: You've gained a solid understanding of what jQuery is and why it's such a popular choice for web development. With its concise syntax and wide range of features, jQuery simplifies complex tasks and boosts productivity.
- DOM Manipulation: jQuery's DOM manipulation capabilities allow you to dynamically modify HTML elements, attributes, and content. Whether you're selecting elements, adding or removing classes, or updating text and HTML, jQuery provides intuitive methods to streamline your workflow.
- Event Handling: Handling user interactions is fundamental to web development. With jQuery, you've learned how to attach event listeners to HTML elements and respond to user actions such as clicks, mouse movements, and keyboard inputs. This enables you to create interactive and engaging web experiences.
- Animation and Effects: jQuery's animation and effects functions enable you to bring your web pages to life with smooth transitions, fades, slides, and more. By animating CSS properties and controlling the timing and easing of animations, you can create visually stunning effects that enhance user engagement.
- Traversing the DOM: Traversing the DOM tree allows you to navigate through HTML elements based on their relationships with each other. With jQuery's traversal methods, you've learned how to efficiently find and select elements, whether they're parents, children, siblings, or descendants.
- Advanced Techniques: You've explored advanced jQuery techniques such as chaining methods, working with dimensions, manipulating CSS styles, and utilizing callback functions. These techniques empower you to create sophisticated web applications and user interfaces.
By mastering jQuery, you've equipped yourself with a powerful toolkit for front-end development. Whether you're building interactive websites, web applications, or mobile apps, jQuery's versatility and efficiency make it an invaluable asset.
Remember, practice makes perfect! Continuously experiment with jQuery, explore its capabilities, and apply your newfound knowledge to real-world projects. Keep learning, stay curious, and embrace the endless possibilities of web development.
Best of luck on your journey as a jQuery developer, and may your future projects shine bright with creativity and innovation!
See the full video:
- jQuery
- JavaScript
- How To Use
- Example Usage
- jQuery Syntax
- jQuery Selectors
- jQuery Events
- Commonly Used Event Methods
- jQuery Effects
- jQuery Callback Function
- jQuery Chaining Methods
- HTML Manipulation
- CSS Manipulation
- jQuery Important Methods
- jQuery Traversing
- Front-End Development
- jQuery Hints
- jQuery Tips